class CrImage::GrayscaleImage
- CrImage::GrayscaleImage
- CrImage::Image
- Reference
- Object
Overview
An image in Grayscale. These image types are the easiest to perform feature and information extraction from, where
there is only one channel to examine, and so has methods for constructing Mask
s from (see #threshold
below).
An RGBAImage
would become a GrayscaleImage
this way:
image.to_gray
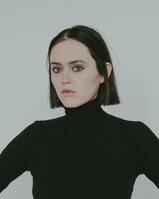
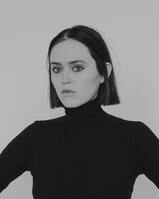
Included Modules
- CrImage::Format::JPEG
- CrImage::Format::PNG
- CrImage::Format::PPM
- CrImage::Format::Save
- CrImage::Format::WebP
- CrImage::Operation::BilinearResize
- CrImage::Operation::BoxBlur
- CrImage::Operation::Brightness
- CrImage::Operation::ChannelSwap
- CrImage::Operation::Contrast
- CrImage::Operation::Crop
- CrImage::Operation::Draw
- CrImage::Operation::GaussianBlur
- CrImage::Operation::HistogramEqualize
- CrImage::Operation::HorizontalBlur
- CrImage::Operation::MaskApply
- CrImage::Operation::Pad
- CrImage::Operation::Rotate
- CrImage::Operation::VerticalBlur
Extended Modules
Defined in:
cr-image/grayscale_image.crjpeg.cr
pluto.cr
png.cr
stumpy.cr
webp.cr
Constructors
-
.from_jpeg(image_data : Bytes) : self
Read
image_data
as JPEG encoded bytes -
.from_jpeg(io : IO) : self
Construct an Image from reading in bytes from
io
-
.from_png(image_data : Bytes) : self
Read
image_data
and PNG encoded bytes -
.from_png(io : IO) : self
Construct an Image by reading bytes from
io
-
.from_ppm(image_data : Bytes) : self
Read
image_data
as PPM encoded bytes -
.from_ppm(io : IO) : self
Read bytes from
io
as PPM encoded -
.from_webp(image_data : Bytes) : self
Read
image_data
as WebP encoded bytes -
.from_webp(io : IO) : self
Read bytes from
io
as WebP encoded -
.new(red : Array(UInt8), green : Array(UInt8), blue : Array(UInt8), alpha : Array(UInt8), width : Int32, height : Int32)
Create a GrayscaleImage from a set of color channels (delegates to
RGBAImage#to_gray
) - .new(gray : Array(UInt8), alpha : Array(UInt8), width : Int32, height : Int32)
-
.new(gray : Array(UInt8), width : Int32, height : Int32)
Create a GrayscaleImage with only an
Array(UInt8)
(alpha channel initialized as255
throughout) -
.new(gray : Array(UInt8), width : Int32)
Create a GrayscaleImage with only an
Array(UInt8)
(alpha channel initialized as255
throughout)
Instance Method Summary
- #<(*args, **options)
- #<(*args, **options, &)
- #<=(arg)
-
#==(arg)
Returns
false
(other can only be aValue
here). - #>(*args, **options)
- #>(*args, **options, &)
- #>=(arg)
-
#[](x : Int32, y : Int32) : Pixel
Return a
Pixel
representing this cell in the image. -
#[](channel_type : ChannelType) : Array(UInt8)
Return the
Array(UInt8)
corresponding tochannel_type
-
#[]=(channel_type : ChannelType, channel : Array(UInt8)) : Array(UInt8)
Set the underlying
Array(UInt8)
ofchannel_type
to the newchannel
. -
#alpha : Array(UInt8)
Return
#alpha
channel - #alpha=(alpha : Array(UInt8))
- #blue : Array(UInt8)
-
#clone : GrayscaleImage
Create a new GrayscaleImage as a copy of this one
- #cross_correlate(map : Map, *, edge_policy : EdgePolicy = EdgePolicy::Repeat) : FloatMap
- #cross_correlate(*args, **options)
- #cross_correlate(*args, **options, &)
- #cross_correlate_fft(map : Map)
-
#each_channel(& : Array(UInt8), ChannelType -> Nil) : Nil
Run provided block with the
ChannelType::Gray
channels and channel types. -
#each_color_channel(& : Array(UInt8), ChannelType -> Nil) : Nil
Run provided block with the
ChannelType::Gray
channels and channel types. - #gray : Array(UInt8)
- #gray=(gray : Array(UInt8))
- #green : Array(UInt8)
-
#height : Int32
Height of image
- #height=(height : Int32)
-
#invert
Invert grayscale pixels (replace each pixel will
255 - p
for allp
in@gray
). -
#invert!
Invert grayscale pixels (replace each pixel will
255 - p
for allp
in@gray
). - #mask_from(*args, **options)
- #mask_from(*args, **options, &)
- #mean(*args, **options)
- #mean(*args, **options, &)
- #red : Array(UInt8)
-
#size : Int32
Return the number of pixels this image contains
-
#threshold(threshold : Int) : Mask
Construct a simple threshold
Mask
containing all pixels with aUInt8
value greater than#threshold
Given sample image: -
#to_a : Array(UInt8)
Receive a copy of the underlying
Array(UInt8)
corresponding to theChannelType::Gray
channel -
#to_gray : GrayscaleImage
Returns self
-
#to_imap : IntMap
Convert this image into a
IntMap
-
#to_map! : UInt8Map
Convert this image into a
UInt8Map
. - #to_pluto : Pluto::ImageGA
-
#to_rgba(color_map : Hash(UInt8, Color), *, default : Color = Color.default) : RGBAImage
Convert this grayscale image to an RGBA one using the provided color map.
-
#to_rgba : RGBAImage
Convert this
GrayscaleImage
to anRGBAImage
. - #to_stumpy : StumpyCore::Canvas
-
#width : Int32
Width of image
- #width=(width : Int32)
Instance methods inherited from module CrImage::Format::WebP
to_webp(io : IO, *, lossy : Bool = false, quality : Int32 = 100) : Nil
to_webp
Instance methods inherited from module CrImage::Format::JPEG
to_jpeg(io : IO, quality : Int32 = 100) : Nil
to_jpeg
Instance methods inherited from module CrImage::Operation::MaskApply
apply(mask : Mask) : selfapply(mask : Mask, &block : UInt8, ChannelType, Int32, Int32 -> UInt8 | Nil) : self apply, apply!(mask : Mask) : self
apply!(mask : Mask, &block : UInt8, ChannelType, Int32, Int32 -> UInt8 | Nil) : self apply!, apply_color(mask : Mask, color : Color) : self apply_color, apply_color!(mask : Mask, color : Color) : self apply_color!
Instance methods inherited from module CrImage::Format::Save
save(filename : String) : self
save
Instance methods inherited from module CrImage::Operation::VerticalBlur
vertical_blur(value : Int32) : self
vertical_blur,
vertical_blur!(value : Int32) : self
vertical_blur!
Instance methods inherited from module CrImage::Operation::Pad
pad(all : Int32 = 0, *, top : Int32 = 0, bottom : Int32 = 0, left : Int32 = 0, right : Int32 = 0, pad_type : EdgePolicy = EdgePolicy::Black) : self
pad,
pad!(all : Int32 = 0, *, top : Int32 = 0, bottom : Int32 = 0, left : Int32 = 0, right : Int32 = 0, pad_type : EdgePolicy = EdgePolicy::Black) : self
pad!
Instance methods inherited from module CrImage::Operation::Rotate
rotate(degrees : Float64, *, center_x : Int32 = width // 2, center_y : Int32 = height // 2, radius : Int32 = -1, pad : Bool = false, pad_type : EdgePolicy = EdgePolicy::Black) : self
rotate,
rotate!(degrees : Float64, *, center_x : Int32 = width // 2, center_y : Int32 = height // 2, radius : Int32 = -1, pad : Bool = false, pad_type : EdgePolicy = EdgePolicy::Black) : self
rotate!
Instance methods inherited from module CrImage::Operation::HorizontalBlur
horizontal_blur(k : Int32) : self
horizontal_blur,
horizontal_blur!(k : Int32) : self
horizontal_blur!
Instance methods inherited from module CrImage::Operation::HistogramEqualize
histogram(channel_type : ChannelType) : Histogram
histogram,
histogram_equalize : self
histogram_equalize,
histogram_equalize! : self
histogram_equalize!
Instance methods inherited from module CrImage::Operation::GaussianBlur
gaussian_blur(sigma : Int32) : self
gaussian_blur,
gaussian_blur!(sigma : Int32) : self
gaussian_blur!
Instance methods inherited from module CrImage::Operation::Draw
draw_circle(x : Int, y : Int, radius : Int, color : Color, *, fill : Bool = false) : selfdraw_circle(region : Region, radius : Int, color : Color, *, fill : Bool = false) : self draw_circle, draw_circle!(center_x : Int, center_y : Int, radius : Int, color : Color, *, fill : Bool = false) : self
draw_circle!(region : Region, color : Color, *, fill : Bool = false, radius : Int32 | Nil = nil) : self draw_circle!, draw_line(x1 : Int32, y1 : Int32, x2 : Int32, y2 : Int32, color : Color) : self draw_line, draw_line!(x1 : Int32, y1 : Int32, x2 : Int32, y2 : Int32, color : Color) : self draw_line!, draw_square(x : Int, y : Int, box_width : Int, box_height : Int, color : Color, *, fill : Bool = false) : self
draw_square(region : Region, color : Color, *, fill : Bool = false) : self draw_square, draw_square!(x : Int, y : Int, box_width : Int, box_height : Int, color : Color, *, fill : Bool = false) : self
draw_square!(region : Region, color : Color, *, fill : Bool = false) : self draw_square!
Instance methods inherited from module CrImage::Operation::Crop
[](xrange : Range, yrange : Range) : self
[],
crop(x : Int32, y : Int32, new_width : Int32, new_height : Int32) : selfcrop(region : Region) : self crop, crop!(x : Int32, y : Int32, new_width : Int32, new_height : Int32) : self
crop!(region : Region) : self crop!
Instance methods inherited from module CrImage::Operation::Contrast
contrast(value : Float64) : self
contrast,
contrast!(value : Float64) : self
contrast!
Instance methods inherited from module CrImage::Operation::ChannelSwap
channel_swap(a : ChannelType, b : ChannelType) : self
channel_swap,
channel_swap!(a : ChannelType, b : ChannelType) : self
channel_swap!
Instance methods inherited from module CrImage::Operation::Brightness
brightness(value : Float64) : self
brightness,
brightness!(value : Float64) : self
brightness!
Instance methods inherited from module CrImage::Operation::BoxBlur
box_blur(value : Int32) : self
box_blur,
box_blur!(value : Int32) : self
box_blur!
Instance methods inherited from module CrImage::Operation::BilinearResize
bilinear_resize(width : Int32, height : Int32) : self
bilinear_resize,
bilinear_resize!(width : Int32, height : Int32) : self
bilinear_resize!
Instance methods inherited from module CrImage::Format::PNG
to_png(io : IO) : Nil
to_png
Instance methods inherited from module CrImage::Format::PPM
to_ppm(io : IO) : Nil
to_ppm
Instance methods inherited from class CrImage::Image
[](channel_type : ChannelType) : Array(UInt8)
[],
[]=(channel_type : ChannelType, channel : Array(UInt8)) : Array(UInt8)
[]=,
alpha : Array(UInt8)
alpha,
blue : Array(UInt8)
blue,
each_channel(& : Array(UInt8), ChannelType -> Nil) : Nil
each_channel,
each_color_channel(& : Array(UInt8), ChannelType -> Nil) : Nil
each_color_channel,
green : Array(UInt8)
green,
height : Int32
height,
red : Array(UInt8)
red,
size : Int32
size,
width : Int32
width
Macros inherited from class CrImage::Image
subsclasses_include(mod)
subsclasses_include
Constructor Detail
Create a GrayscaleImage from a set of color channels (delegates to RGBAImage#to_gray
)
Create a GrayscaleImage with only an Array(UInt8)
(alpha channel initialized as 255
throughout)
Create a GrayscaleImage with only an Array(UInt8)
(alpha channel initialized as 255
throughout)
Instance Method Detail
Returns false
(other can only be a Value
here).
Return a Pixel
representing this cell in the image.
Return the Array(UInt8)
corresponding to channel_type
Set the underlying Array(UInt8)
of channel_type
to the new channel
.
Warning: this method does not check the size of the incoming array, and if it's a different
size from what the current image represents, this could break it. We recommend against using
this method except for from other methods that will be updating the #width
and #height
immediately after.
Run provided block with the ChannelType::Gray
channels and channel types.
Run provided block with the ChannelType::Gray
channels and channel types.
Invert grayscale pixels (replace each pixel will 255 - p
for all p
in @gray
). Modifies self.
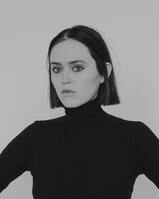
Becomes
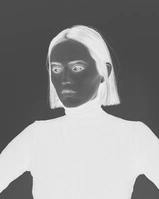
Construct a simple threshold Mask
containing all pixels with a UInt8
value greater than #threshold
Given sample image:
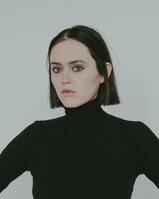
image
.to_gray # convert color image to grayscale one
.threshold(128) # generate a mask using threshold operator
.to_gray # convert mask to grayscale image
.save("threshold_example.jpg") # save mask as grayscale
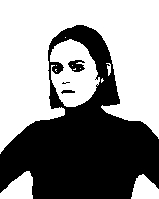
Receive a copy of the underlying Array(UInt8)
corresponding to the ChannelType::Gray
channel
Convert this image into a UInt8Map
. Does not clone underlying #gray
channel, both this image and map share the same data.
Convert this grayscale image to an RGBA one using the provided color map.
The provided map should have a key for all 0-255 possible gray pixel values, otherwise the default
Color
will be used instead (default is black).
colors = 256.times.to_a.map { |i| {i.to_u8, CrImage::Color.random} }.to_h
gray_image.to_rgba(colors).save("to_rgba_color_map_sample.jpg")
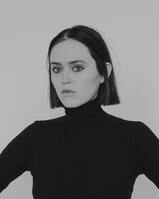
Becomes
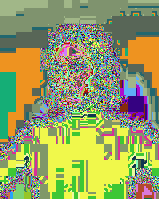
Convert this GrayscaleImage
to an RGBAImage
.
No color will be provided, all pixels will remain gray.