module CrImage::MapImpl(T)
Overview
A collection of implementations of NumericMap(T)
, intended to be included in base classes.
See IntMap
, FloatMap
, and ComplexMap
.
Included Modules
Direct including types
Defined in:
cr-image/map.crConstructors
- .new(width : Int32, height : Int32, initial : T)
- .new(width : Int32, raw : Array(T))
- .new(other : Array(Array(T)))
- .new(width : Int32, height : Int32, &)
Instance Method Summary
-
#*(num : Int | Float) : self
Multiply each point in this
Map
bynum
-
#+(num : Int | Float) : self
Add each point in this
Map
bynum
-
#+(other : self) : self
Add each point of this
Map
with each point inother
- #-(other : NumericMap(T)) : self
-
#-(num : Int) : self
Subtract each point in this
Map
bynum
-
#-(num : Float) : FloatMap
Subtract each point in this
Map
bynum
-
#/(num : Int | Float) : FloatMap
Divides each point in this
Map
bynum
-
#<(num : Int | Float) : Mask
Construct a
Mask
identifying all pixels smaller thannum
. -
#<=(num : Int | Float) : Mask
Construct a
Mask
identifying all pixels smaller than or equal tonum
. -
#==(num : Int | Float) : Mask
Construct a
Mask
identifying all pixels equal tonum
-
#==(other : self) : Bool
Check if this
Map
is equal toother
-
#>(num : Int | Float) : Mask
Construct a
Mask
identifying all pixels larger thannum
. -
#>=(num : Int | Float) : Mask
Construct a
Mask
identify all pixels larger than or equal tonum
. -
#[](xstart : Int32, xcount : Int32, ystart : Int32, ycount : Int32) : self
Crop the map to the values contained from
xstart
out byxcount
, andystart
out byycount
-
#[](x : Int32, y : Int32) : T
Get element at coordinates
x
andy
-
#[](xrange : Range, ystart : Int32) : self
Crop the map, returning only the values in row at
ystart
-
#[](xstart : Int32, yrange : Range) : self
Crop the map, returning only the values in column at
xstart
-
#[](xrange : Range, yrange : Range) : self
Crop the map to the values contained in
xrange
andyrange
-
#[](index : Int32) : T
Get element at
index
from underlying#raw
Array. -
#[]?(x : Int32, y : Int32) : T | Nil
Get lement at coordinates
x
andy
, ornil
if coordinates are out of bounds -
#[]?(index : Int32) : T | Nil
Get element at
index
, ornil
if out of bounds -
#column(x : Int32) : self
Get a single dimensional
Map
representing teh column atx
-
#cross_correlate(template : Map, *, edge_policy : EdgePolicy = EdgePolicy::Repeat) : FloatMap
Perform a brute force cross correlation calculation with provided
template
. -
#cross_correlate_fft(template : Map, *, edge_policy : EdgePolicy = EdgePolicy::Black) : FloatMap
Perform a Fast Fourier Transform cross correlation (convolution?) with provided
template
. -
#fft : ComplexMap
Performe a Fast Fourier Transform, padding out this
Map
so dimensions are a power of 2. - #height : Int32
-
#mask_from(&block : T, Int32, Int32 -> Bool) : Mask
Construct a
Mask
from thisGrayscaleImage
using the passed in block to determine if a given pixel should be true or not -
#max : T
Return the maximum value in this
Map
-
#mean : Float64
Return the average of the elements in this
Map
-
#min : T
Return the minimum value in this
Map
-
#pad(all : Int32 = 0, *, top : Int32 = 0, bottom : Int32 = 0, left : Int32 = 0, right : Int32 = 0, pad_type : EdgePolicy = EdgePolicy::Black, pad_black_value : T = T.zero) : self
Pad the borders of this
Map
corresponding topad_type
. -
#raw : Array(T)
Return the raw array underlying the map
-
#row(y : Int32) : self
Get a single dimensional
Map
representing the row aty
-
#shape : Tuple(Int32, Int32)
Return the shape of the map -
{width, height}
-
#size : Int32
Size of the map -
width * height
-
#sum : T
Return the sum of all values in this
Map
-
#to_a : Array(T)
Receive a copy of the underlying
#raw
array. -
#to_c : ComplexMap
Convert this
Map
to aComplexMap
-
#to_gray(*, scale : Bool = false) : GrayscaleImage
Convert this
Map
to aGrayscaleImage
. - #width : Int32
Instance methods inherited from module CrImage::NumericMap(T)
+(other : NumericMap(T)) : NumericMap(T)
+,
-(other : NumericMap(T)) : NumericMap(T)
-,
max : T
max,
min : T
min,
sum : T
sum,
to_c : NumericMap(Complex)
to_c,
to_f : NumericMap(Float64)
to_f,
to_i : NumericMap(Int32)
to_i
Instance methods inherited from module CrImage::Map(T)
[](x : Int32, y : Int32) : T[](index : Int32) : T [], []?(x : Int32, y : Int32) : T | Nil
[]?(index : Int32) : T | Nil []?, height : Int32 height, shape : Tuple(Int32, Int32) shape, size : Int32 size, width : Int32 width
Constructor Detail
Instance Method Detail
Divides each point in this Map
by num
TODO ComplexMap shouldn't return a FloatMap
Construct a Mask
identifying all pixels smaller than num
.
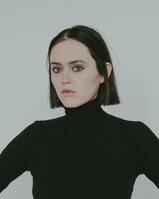
gray = image.to_gray # Convert color image to grayscale
mask = gray < 128 # Generate a threshold mask
mask.to_gray.save("greater_than_example.jpg") # Convert and save the mask as a black and white image
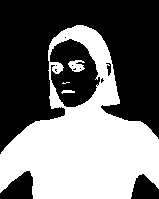
Construct a Mask
identifying all pixels smaller than or equal to num
. See #<
for example.
Construct a Mask
identifying all pixels equal to num
Construct a Mask
identifying all pixels larger than num
.
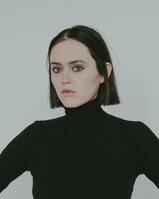
gray = image.to_gray # Convert color image to grayscale
mask = gray > 128 # Generate a threshold mask
mask.to_gray.save("greater_than_example.jpg") # Convert and save the mask as a black and white image
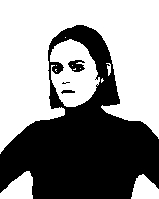
Construct a Mask
identify all pixels larger than or equal to num
. See #>
for near example.
Crop the map to the values contained from xstart
out by xcount
, and ystart
out by ycount
Crop the map, returning only the values in row at ystart
Crop the map, returning only the values in column at xstart
Crop the map to the values contained in xrange
and yrange
Get lement at coordinates x
and y
, or nil
if coordinates are out of bounds
Perform a brute force cross correlation calculation with provided template
.
Perform a Fast Fourier Transform cross correlation (convolution?) with provided template
. See FftUtil
Construct a Mask
from this GrayscaleImage
using the passed in block to determine if a given pixel should be true or not
# Construct a mask identifying the bright pixels in the bottom left corner of image
image.to_gray.mask_from do |pixel, x, y|
x < image.width // 2 && # left half of image
y > (image.height // 2) && # bottom half of image
pixel > 128 # only "bright" pixels
end
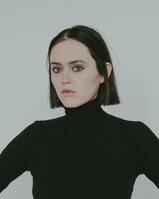
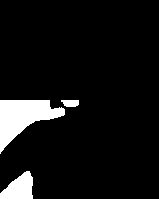
Pad the borders of this Map
corresponding to pad_type
.
Convert this Map
to a GrayscaleImage
.
Set scale: true
so that all of the values will scale, such that #max
will become 255u8
, and #min
will become 0u8
. All other values will be
linearly scaled between those values.